Path Manifests
Path manifests map paths to transaction IDs. Use them to create logical groupings of transactions, even transactions you didn’t create yourself.
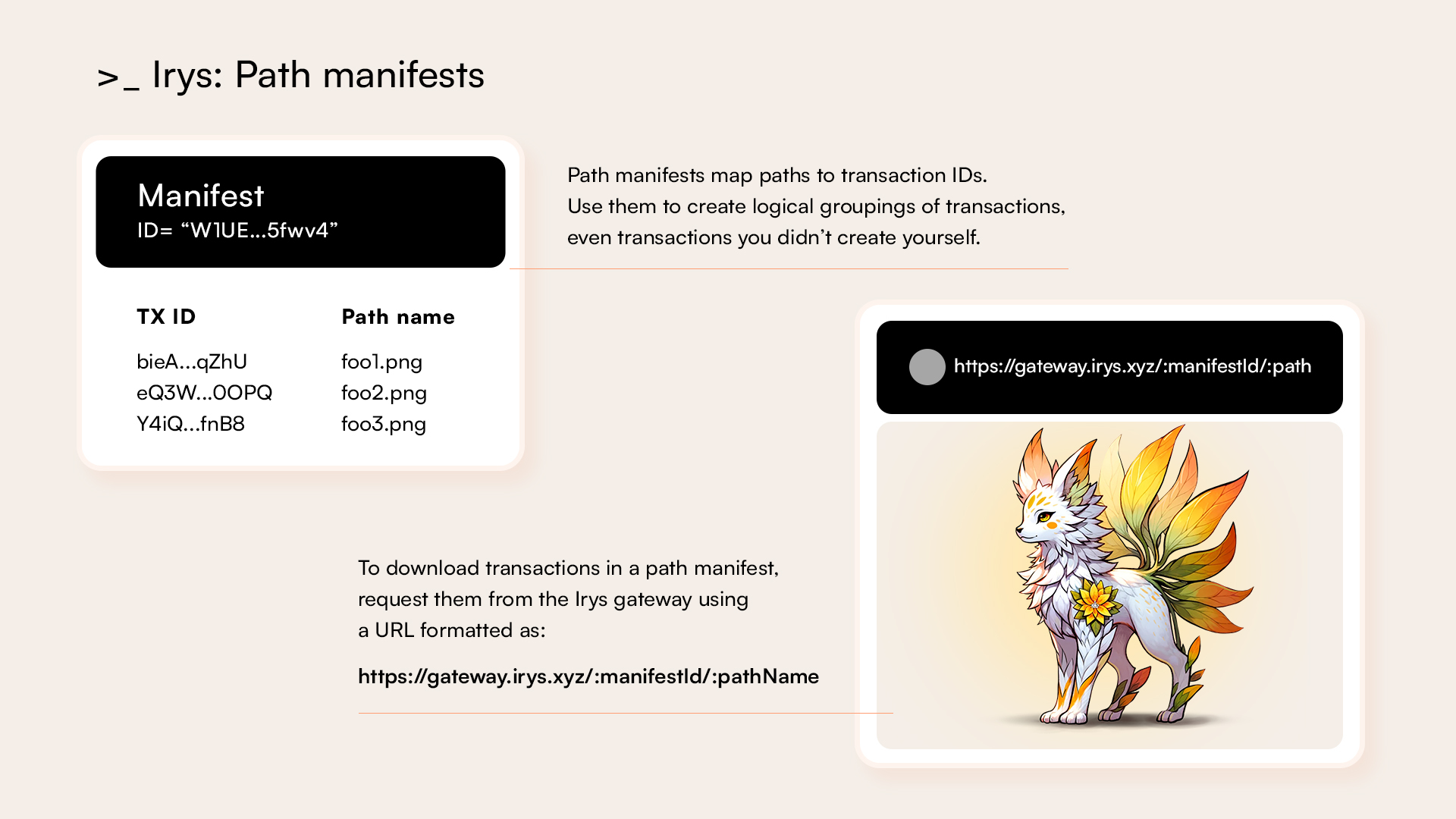
Creating
To create a path manifest:
- Create a JavaScript
Map
object where each entry maps a unique transaction ID to a unique path. Paths are arbitrary; you can use anything that conforms to valid URL syntax. - Create a
Manifest
object by passing theMap
object toirys.uploader.generateManifest()
. - Upload the
Manifest
object to Irys.
const map = new Map();
map.set("foo.png", "DSfHGmnhb7AN3xY3VUCykl-2xKiQcqXrsSP9zpzQQmY");
const manifest = await irys.uploader.generateManifest({ items: map });
const tags = [
{ name: "Type", value: "manifest" },
{ name: "Content-Type", value: "application/x.irys-manifest+json" },
];
const receipt = await irys.upload(JSON.stringify(manifest), { tags });
const manifestId = receipt.id;
Resolving
Upon uploading a manifest, you get a receipt containing a manifest ID. To download transactions in a path manifest, request them from the Irys gateway using a URL formatted as:
https://gateway.irys.xyz/[manifestId]/[pathName]
The gateway then:
- Looks up the manifest by ID.
- Looks in the manifest to see if the path exists.
- Returns the transaction associated with the path if found.
- Returns 404 if not found.
For example, if you have a manifest with ID W1UbYAZ08egXgm9_kCw24ZZPAfdu8LQB7jc_Vx8fwv4
containing the following:
Tx ID | Path Name |
---|---|
DSfHGmnhb7AN3xY3VUCykl-2xKiQcqXrsSP9zpzQQmY | foo1.png |
JDCzc3RE5b6RBXt3foKOR_nTt76dIxoW3Jjjezkk6VA | foo2.png |
7BaKT3Wm04NPEAL3A0jcRc4cwQ6KHV8krv-DkbneFBw | foo3.png |
You can download the first entry using:
https://gateway.irys.xyz/W1UbYAZ08egXgm9_kCw24ZZPAfdu8LQB7jc_Vx8fwv4/foo1.png
Manually Creating a Path Manifest
Use path manifests to create a logical grouping of transactions, even transactions you didn’t create yourself.
This example:
- Uses 10 existing transaction IDs.
- Groups the transactions using a
Map
object. - Creates a manifest mapping the transactions to paths.
- Permanently uploads the manifest using Irys.
// Get the most recent 'totalIds' number TXs tagged 'image/gif'
const getTxIds = async () => {
const txIds = [
'DSfHGmnhb7AN3xY3VUCykl-2xKiQcqXrsSP9zpzQQmY',
'vW3jH4kpL8mT9rXsUo7dE1cXfP5qY1kJzG2j6V8a3n9',
'9cJ5KzY8rV2mL0N1Xg4T7wBqP3hD6fFz0o2v9u3E5i1',
'P3jH8mT5r0N2L6Xy7K1zQ4oV9cE2fFzJ5w8u0d3i1g6',
'r9X0L4T2m5K8P1H6V7o3zYQfE9wC2j0i3u5D6F7n8g',
'K2mL7T5r1X8P0H9V3zY4oE6fFzJ2w8u0d3i1g9n5C7',
'T5r8X0L4m1P2H7V9o3zY6fE2fJ0wC5u3i1g9D7n8K',
'6V7K0T5r1X2P8H9m3zY4oE2fFzJ5w8u0d1i9g7n3C',
'm1X2T7P5r8H9V0o3zY4fE6fFzJ2w8u0d3i1g9n7C',
'P8L7m1T0X2H9V3o4zY5E6fFzJ2w8u0d3i1g9n7K8C'
];
return txIds;
};
// Generate a manifest containing 10 Tx IDs
const generateManifest = async () => {
const txIds: string[] = getTxIds();
const map = new Map();
for (let i = 0; i < txIds.length; i++) {
map.set(`foo${i}.gif`, txIds[i]);
}
const irys = await getIrys();
const manifest = await irys.uploader.generateManifest({ items: map });
const tags = [
{ name: "Type", value: "manifest" },
{ name: "Content-Type", value: "application/x.irys-manifest+json" },
];
const receipt = await irys.upload(JSON.stringify(manifest), { tags });
const manifestId = receipt.id;
map.forEach((value, key) => {
console.log(`https://gateway.irys.xyz/${manifestId}/${key}`);
});
};
async function main(): Promise<void> {
await generateManifest();
}
main().catch(console.error);